在這篇文章中,我們將深入探討如何在C#中使用HttpClient.PostAsync
進行HTTP POST請求。我們將涵蓋基礎知識、一些高級用法以及實際應用示例。讓我們開始吧!
什么是HttpClient?
HttpClient
是.NET庫中的一個類,用于處理HTTP請求。它可以讓你發送數據到服務器或從服務器獲取數據。
使用HttpClient的優勢
異步操作:確保你的應用在等待響應時不會凍結。
可重用:你可以使同一個實例進行多次請求。
靈活性:支持多種HTTP方法和可定制的頭信息。
HttpClient.PostAsync的定義和用途
HttpClient.PostAsync
基本上是告訴你的程序使用HTTP POST方法異步地向指定的URL發送數據。想象一下,它就像是即時可靠地郵寄一封信。
何時使用PostAsync
當你需要向服務器發送數據以創建或更新資源時,使用PostAsync
。這就像提交表單或上傳文件。
基本語法和用法
以下是一個簡單的示例:
HttpClient client = new HttpClient();
User user = new User();
user.name = "張三";
user.city = "北京";
string jsonData = System.Text.Json.JsonSerializer.Serialize(user);
HttpContent content = new StringContent(jsonData, Encoding.UTF8, "application/json");
HttpResponseMessage response = await client.PostAsync("https://localhost:7144/api/User/Add", content);
Console.WriteLine(await response.Content.ReadAsStringAsync());
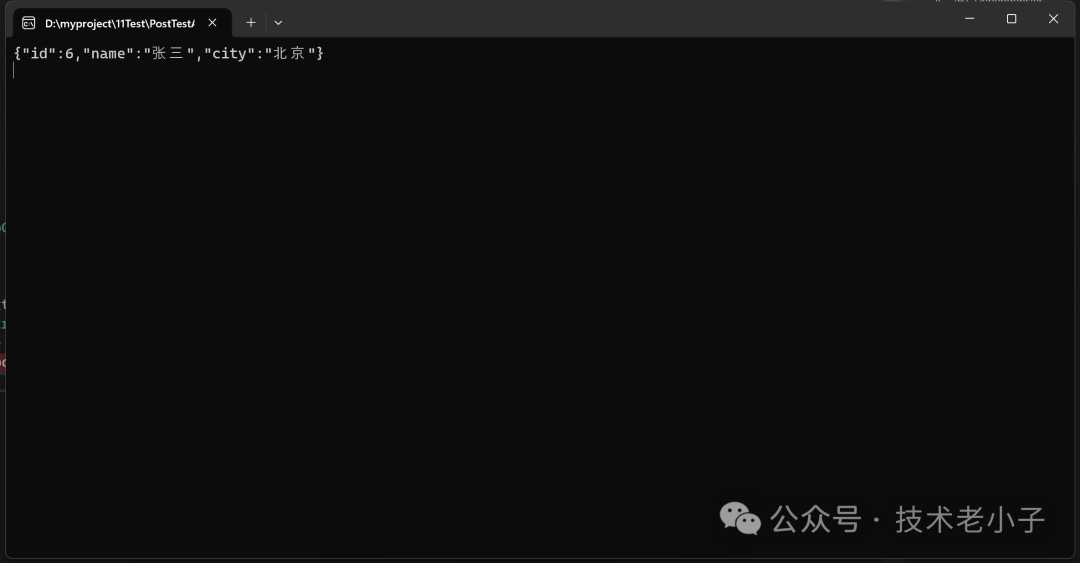
在這個代碼片段中,jsonData
是你的JSON格式的數據。這就像是在發送包裹前進行包裝。
如何執行基本的POST請求
發送簡單數據
讓我們動手實踐一下,以下是如何發送簡單的字符串數據:
var client = new HttpClient();
var content = new StringContent("This is some data", Encoding.UTF8, "text/plain");
HttpResponseMessage response = await client.PostAsync("https://localhost:7144/api/User/post", content);
Console.WriteLine(await response.Content.ReadAsStringAsync());
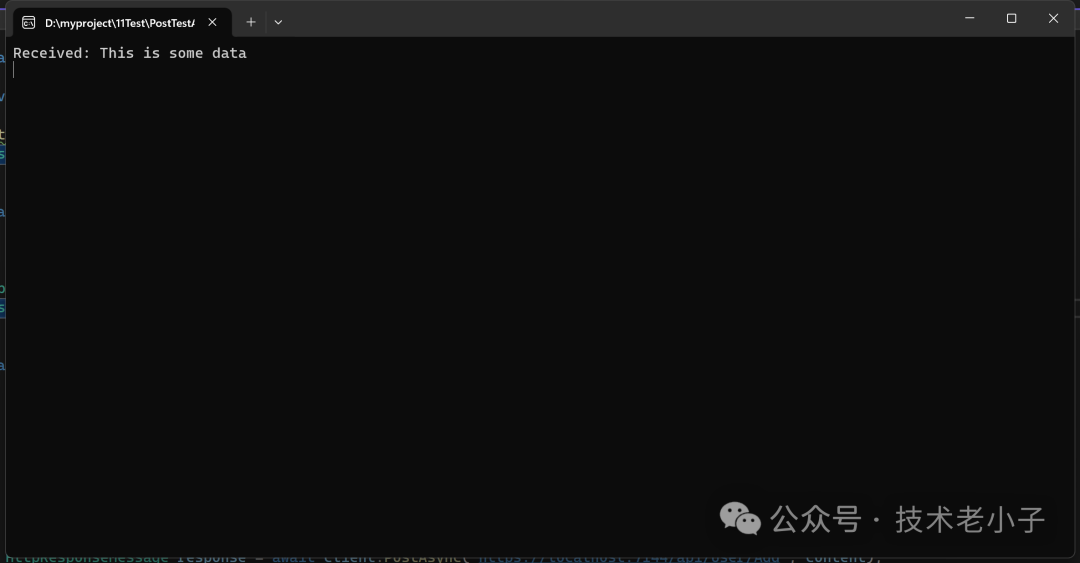
處理表單數據
有時,你需要發送表單數據,就像在網頁上提交表單一樣。以下是操作方法:
HttpClient client = new HttpClient();
var form = new MultipartFormDataContent();
form.Add(new StringContent("John"), "name");
form.Add(new StringContent("New York"), "city");
var response = await client.PostAsync("https://localhost:7144/api/User/Post", form);
Console.WriteLine(await response.Content.ReadAsStringAsync());
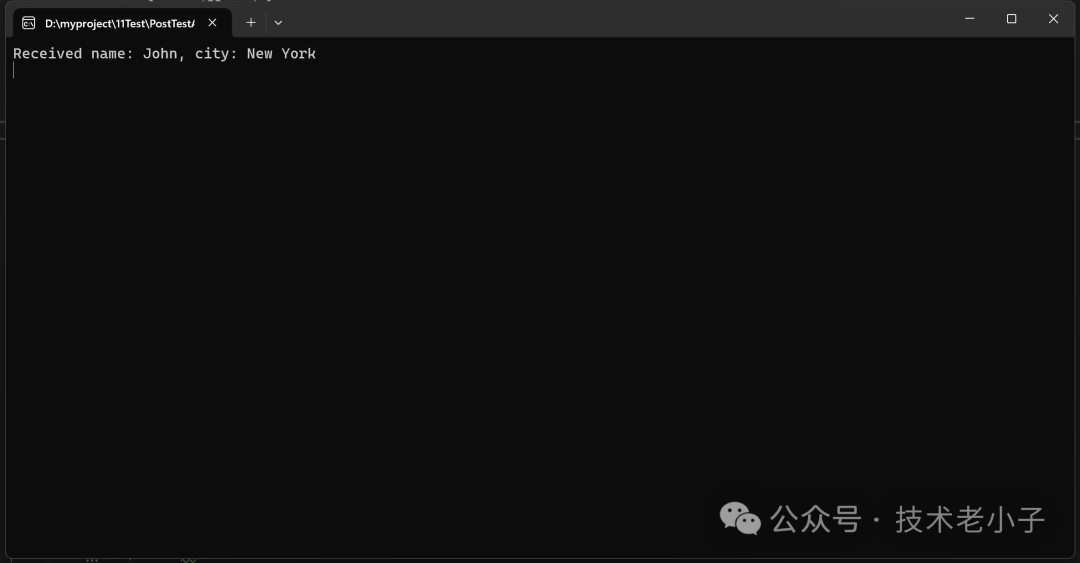
HttpClient.PostAsync的高級用法
添加請求頭
請求頭就像包裹上的標簽,提供額外的元數據:
var client = new HttpClient();
client.DefaultRequestHeaders.Add("Authorization", "Bearer?YOUR_TOKEN_HERE");
使用身份驗證
當你需要證明你是誰時,使用身份驗證。以下是一個基本示例:
var client = new HttpClient();
var byteArray = Encoding.ASCII.GetBytes("username:password");
client.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Basic", Convert.ToBase64String(byteArray));
處理不同的內容類型
除了JSON,你可能需要處理XML或其他格式:
HttpContent content = new StringContent("<xml><name>John</name></xml>", Encoding.UTF8, "application/xml");
HttpResponseMessage response = await client.PostAsync("https://example.com/api", content);
錯誤處理
常見錯誤和異常
錯誤處理最佳實踐
始終檢查響應狀態。以下是高效捕獲錯誤的方法:
try
{
var response = await client.PostAsync(uri, content);
response.EnsureSuccessStatusCode();
}
catch (HttpRequestException e)
{
Console.WriteLine($"Request error: {e.Message}");
}
性能優化
高效的連接管理
重用HttpClient
實例以優化性能:
static readonly HttpClient client = new HttpClient();
優化高吞吐量
對于高容量請求,最小化阻塞:
var tasks = new List<Task<HttpResponseMessage>>();
foreach (var item in items)
{
tasks.Add(client.PostAsync(uri, new StringContent(item)));
}
await Task.WhenAll(tasks);
異步編程最佳實踐
異步方法應正確等待以避免死鎖。
實際應用示例
創建一個REST客戶端
假設你想創建一個可重用的REST客戶端。以下是骨架代碼:
public class RestClient
{
private static readonly HttpClient client = new HttpClient();
public async Task<string> PostDataAsync(string uri, string jsonData)
{
var content = new StringContent(jsonData, Encoding.UTF8, "application/json");
var response = await client.PostAsync(uri, content);
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
}
RestClient client = new RestClient();
User user = new User();
user.name = "張三";
user.city = "北京";
string jsonData = System.Text.Json.JsonSerializer.Serialize(user);
string ret = await client.PostDataAsync("https://localhost:7144/api/User/Add",jsonData);
Console.WriteLine(ret);
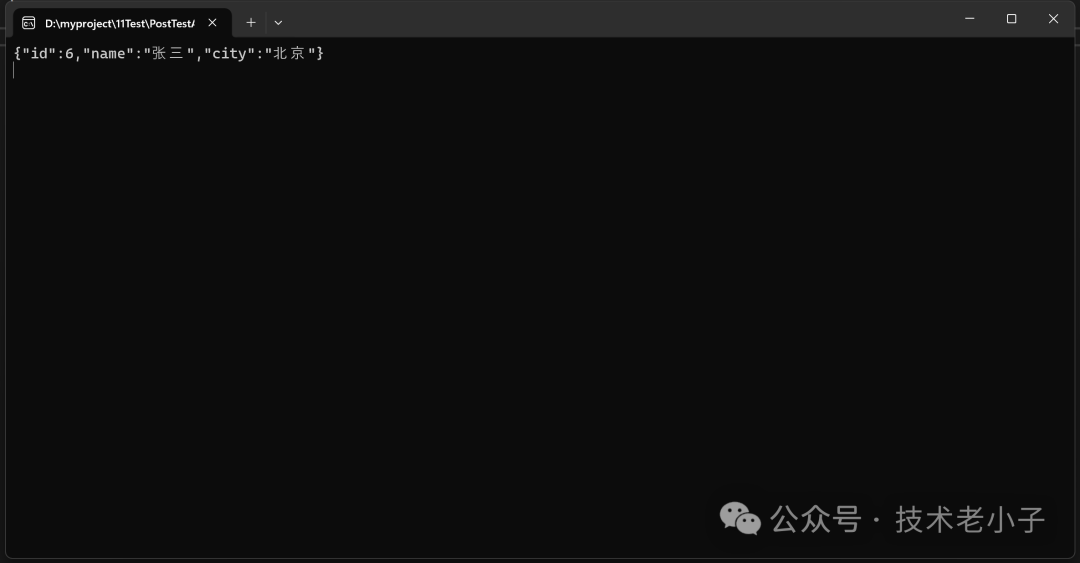
上傳文件
需要上傳文件?以下是操作方法:
var form = new MultipartFormDataContent();
var fileContent = new ByteArrayContent(File.ReadAllBytes("filePath"));
form.Add(fileContent, "file", "fileName.txt");
var response = await client.PostAsync("https://example.com/upload", form);
故障排除
調試常見問題
問題可能會讓你困惑,但像Fiddler和Postman這樣的工具可以拯救你。它們允許你檢查HTTP請求和響應。
故障排除工具和技術
結論
HttpClient.PostAsync
是一個在C#中進行POST請求的強大工具。它簡單、靈活且功能強大。從簡單的數據提交到復雜的身份驗證請求,HttpClient
都能勝任。探索這個多功能工具將為你的C#項目打開新的可能性,使你的網絡交互更加順暢和高效。
該文章在 2024/7/31 12:43:00 編輯過