1. 簡介
WebSocket-sharp 是一個功能強(qiáng)大的 C# WebSocket 庫,支持 WebSocket 客戶端和服務(wù)器端的實(shí)現(xiàn)。它具有以下主要特性:
完全支持 RFC 6455 協(xié)議規(guī)范
支持客戶端和服務(wù)器端實(shí)現(xiàn)
支持消息壓縮擴(kuò)展
支持安全連接(SSL/TLS)
支持 HTTP 認(rèn)證
支持查詢字符串、Origin 頭和 Cookies
支持 HTTP 代理服務(wù)器連接
支持 .NET Framework 3.5 及更高版本
2. 安裝方式
2.1 手動構(gòu)建
使用 MonoDevelop 打開 websocket-sharp.sln
構(gòu)建 websocket-sharp 項(xiàng)目
將生成的 websocket-sharp.dll 添加到你的項(xiàng)目引用中
2.2 NuGet 安裝
使用 NuGet Package Manager Console:
PM>?Install-Package?WebSocketSharp.core
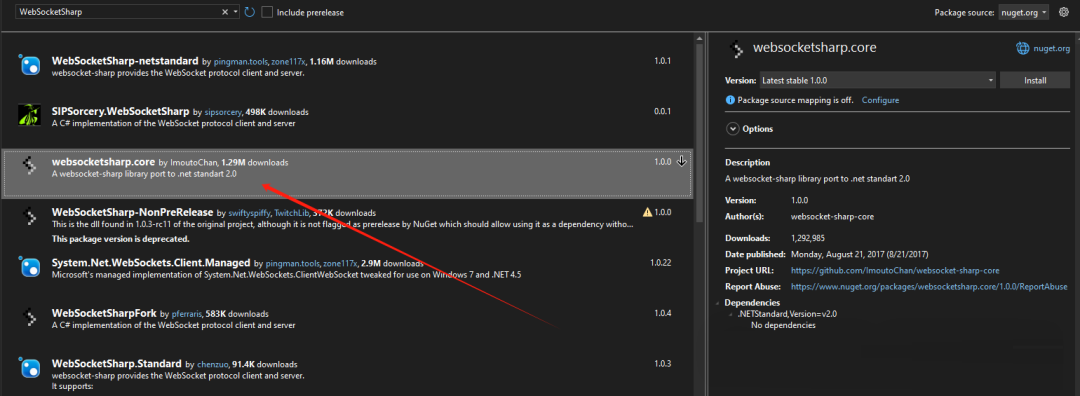
3. 客戶端實(shí)現(xiàn)
3.1 基礎(chǔ)客戶端示例
internal?class?Program
{
? ?static?void?Main()
? ?{
? ? ? ?using?(var?ws?=?new?WebSocket("ws://127.0.0.1:4649/echo"))
? ? ? ?{
? ? ? ? ? ?//?連接建立時的處理 ?
? ? ? ? ? ?ws.OnOpen?+=?(sender,?e)?=>?{
? ? ? ? ? ? ? ?Console.WriteLine("連接已建立");
? ? ? ? ? ?};
? ? ? ? ? ?//?接收消息的處理 ?
? ? ? ? ? ?ws.OnMessage?+=?(sender,?e)?=>?{
? ? ? ? ? ? ? ?Console.WriteLine($"收到服務(wù)器消息:?{e.Data}");
? ? ? ? ? ?};
? ? ? ? ? ?//?發(fā)生錯誤時的處理 ?
? ? ? ? ? ?ws.OnError?+=?(sender,?e)?=>?{
? ? ? ? ? ? ? ?Console.WriteLine($"發(fā)生錯誤:?{e.Message}");
? ? ? ? ? ?};
? ? ? ? ? ?//?連接關(guān)閉時的處理 ?
? ? ? ? ? ?ws.OnClose?+=?(sender,?e)?=>?{
? ? ? ? ? ? ? ?Console.WriteLine($"連接關(guān)閉:?{e.Code}?{e.Reason}");
? ? ? ? ? ?};
? ? ? ? ? ?try
? ? ? ? ? ?{
? ? ? ? ? ? ? ?//?連接到服務(wù)器 ?
? ? ? ? ? ? ? ?ws.Connect();
? ? ? ? ? ? ? ?//?等待連接建立 ?
? ? ? ? ? ? ? ?Thread.Sleep(1000);
? ? ? ? ? ? ? ?if?(ws.ReadyState?==?WebSocketState.Open)
? ? ? ? ? ? ? ?{
? ? ? ? ? ? ? ? ? ?Console.WriteLine("正在發(fā)送消息...");
? ? ? ? ? ? ? ? ? ?ws.Send("Hello?Server!");
? ? ? ? ? ? ? ? ? ?//?保持連接一段時間以接收響應(yīng) ?
? ? ? ? ? ? ? ? ? ?Thread.Sleep(1000);
? ? ? ? ? ? ? ?}
? ? ? ? ? ? ? ?else
? ? ? ? ? ? ? ?{
? ? ? ? ? ? ? ? ? ?Console.WriteLine("連接未就緒,當(dāng)前狀態(tài):?"?+?ws.ReadyState);
? ? ? ? ? ? ? ?}
? ? ? ? ? ? ? ?Console.WriteLine("按任意鍵退出...");
? ? ? ? ? ? ? ?Console.ReadKey(true);
? ? ? ? ? ?}
? ? ? ? ? ?catch?(Exception?ex)
? ? ? ? ? ?{
? ? ? ? ? ? ? ?Console.WriteLine($"發(fā)生異常:?{ex.Message}");
? ? ? ? ? ?}
? ? ? ?}
? ?}
}
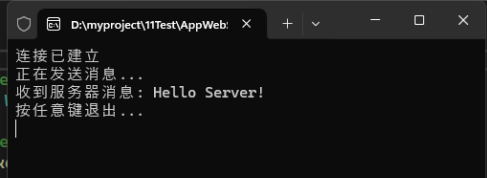
3.2 帶安全連接的客戶端示例
using?System.Security.Cryptography.X509Certificates;
var?ws?=?new?WebSocket("wss://example.com");
//?設(shè)置服務(wù)器證書驗(yàn)證
ws.SslConfiguration.ServerCertificateValidationCallback?=?
? ?(sender,?certificate,?chain,?sslPolicyErrors)?=>?{
? ? ? ?//?在這里進(jìn)行證書驗(yàn)證邏輯
? ? ? ?return?true;?//?返回?true?表示證書有效
? ?};
3.3 帶認(rèn)證的客戶端示例
var?ws?=?new?WebSocket("ws://example.com");
ws.SetCredentials("username",?"password",?true);
4. 服務(wù)器端實(shí)現(xiàn)
4.1 基礎(chǔ)服務(wù)器示例
public?class?Echo?:?WebSocketBehavior
{
? ?protected?override?void?OnOpen()
? ?{
? ? ? ?Console.WriteLine("客戶端已連接");
? ?}
? ?protected?override?void?OnMessage(MessageEventArgs?e)
? ?{
? ? ? ?Console.WriteLine($"服務(wù)器收到消息:?{e.Data}");
? ? ? ?Send(e.Data);?//?回顯消息 ?
? ?}
? ?protected?override?void?OnClose(CloseEventArgs?e)
? ?{
? ? ? ?Console.WriteLine("客戶端已斷開連接");
? ?}
? ?protected?override?void?OnError(ErrorEventArgs?e)
? ?{
? ? ? ?Console.WriteLine($"服務(wù)器端錯誤:?{e.Message}");
? ?}
}
class?Program
{
? ?static?void?Main()
? ?{
? ? ? ?//?創(chuàng)建?WebSocket?服務(wù)器 ?
? ? ? ?var?wssv?=?new?WebSocketServer("ws://0.0.0.0:4649");
? ? ? ?//?添加?WebSocket?服務(wù) ?
? ? ? ?wssv.AddWebSocketService<Echo>("/echo");
? ? ? ?//?啟動服務(wù)器 ?
? ? ? ?wssv.Start();
? ? ? ?Console.WriteLine("WebSocket?服務(wù)器已啟動在?ws://0.0.0.0:4649/echo");
? ? ? ?Console.WriteLine("按任意鍵停止服務(wù)器...");
? ? ? ?Console.ReadKey(true);
? ? ? ?//?停止服務(wù)器 ?
? ? ? ?wssv.Stop();
? ?}
}
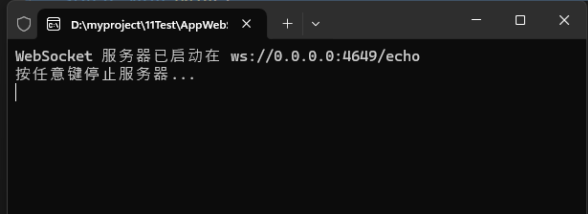
4.2 聊天室服務(wù)器示例
using?WebSocketSharp.Server;
using?WebSocketSharp;
using?ErrorEventArgs?=?WebSocketSharp.ErrorEventArgs;
namespace?AppChatServer
{
? ?//?聊天室處理類 ?
? ?publicclass?ChatRoom?:?WebSocketBehavior
? ?{
? ? ? ?privatestatic?Dictionary<string,?string>?_users?=?new?Dictionary<string,?string>();
? ? ? ?privatestring?_nickname;
? ? ? ?protected?override?void?OnOpen()
? ? ? ?{
? ? ? ? ? ?//?從?Context.QueryString?獲取昵稱 ?
? ? ? ? ? ?var?queryString?=?Context.QueryString;
? ? ? ? ? ?_nickname?=?queryString["nickname"]????$"Anonymous_{GetRandomString(4)}";
? ? ? ? ? ?lock?(_users)
? ? ? ? ? ?{
? ? ? ? ? ? ? ?_users[ID]?=?_nickname;
? ? ? ? ? ?}
? ? ? ? ? ?Console.WriteLine($"新用戶加入:?{_nickname}");
? ? ? ? ? ?Sessions.Broadcast($"{_nickname}?加入了聊天室");
? ? ? ? ? ?//?發(fā)送當(dāng)前在線用戶列表 ?
? ? ? ? ? ?var?userList?=?string.Join(",?",?_users.Values);
? ? ? ? ? ?Sessions.Broadcast($"當(dāng)前在線用戶:?{userList}");
? ? ? ?}
? ? ? ?protected?override?void?OnMessage(MessageEventArgs?e)
? ? ? ?{
? ? ? ? ? ?Console.WriteLine($"收到消息?from?{_nickname}:?{e.Data}");
? ? ? ? ? ?Sessions.Broadcast($"{_nickname}:?{e.Data}");
? ? ? ?}
? ? ? ?protected?override?void?OnClose(CloseEventArgs?e)
? ? ? ?{
? ? ? ? ? ?lock?(_users)
? ? ? ? ? ?{
? ? ? ? ? ? ? ?_users.Remove(ID);
? ? ? ? ? ?}
? ? ? ? ? ?Console.WriteLine($"用戶離開:?{_nickname}");
? ? ? ? ? ?Sessions.Broadcast($"{_nickname}?離開了聊天室");
? ? ? ? ? ?//?更新在線用戶列表 ?
? ? ? ? ? ?var?userList?=?string.Join(",?",?_users.Values);
? ? ? ? ? ?Sessions.Broadcast($"當(dāng)前在線用戶:?{userList}");
? ? ? ?}
? ? ? ?protected?override?void?OnError(ErrorEventArgs?e)
? ? ? ?{
? ? ? ? ? ?Console.WriteLine($"發(fā)生錯誤?({_nickname}):?{e.Message}");
? ? ? ?}
? ? ? ?private?string?GetRandomString(int?length)
? ? ? ?{
? ? ? ? ? ?conststring?chars?=?"ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
? ? ? ? ? ?Random?random?=?new?Random();
? ? ? ? ? ?returnnewstring(Enumerable.Repeat(chars,?length)
? ? ? ? ? ? ? ?.Select(s?=>?s[random.Next(s.Length)]).ToArray());
? ? ? ?}
? ?}
? ?//?服務(wù)器主程序 ?
? ?class?Program
? ?{
? ? ? ?static?void?Main()
? ? ? ?{
? ? ? ? ? ?//?創(chuàng)建?WebSocket?服務(wù)器 ?
? ? ? ? ? ?var?wssv?=?new?WebSocketServer("ws://0.0.0.0:4649");
? ? ? ? ? ?//?添加聊天室服務(wù) ?
? ? ? ? ? ?wssv.AddWebSocketService<ChatRoom>("/chat");
? ? ? ? ? ?//?啟動服務(wù)器 ?
? ? ? ? ? ?wssv.Start();
? ? ? ? ? ?Console.WriteLine("聊天室服務(wù)器已啟動在?ws://0.0.0.0:4649/chat");
? ? ? ? ? ?Console.WriteLine("按任意鍵停止服務(wù)器...");
? ? ? ? ? ?Console.ReadKey(true);
? ? ? ? ? ?//?停止服務(wù)器 ?
? ? ? ? ? ?wssv.Stop();
? ? ? ? ? ?Console.WriteLine("服務(wù)器已停止");
? ? ? ?}
? ?}
}
客戶端
using?WebSocketSharp;
namespace?AppChatClient
{
? ?class?Program
? ?{
? ? ? ?static?void?Main()
? ? ? ?{
? ? ? ? ? ?Console.Write("請輸入你的昵稱:?");
? ? ? ? ? ?string?nickname?=?Console.ReadLine()?.Trim()????"Anonymous";
? ? ? ? ? ?//?創(chuàng)建WebSocket連接,包含昵稱參數(shù) ?
? ? ? ? ? ?using?(var?ws?=?new?WebSocket($"ws://127.0.0.1:4649/chat?nickname={Uri.EscapeDataString(nickname)}"))
? ? ? ? ? ?{
? ? ? ? ? ? ? ?bool?isConnected?=?false;
? ? ? ? ? ? ? ?//?連接建立時的處理 ?
? ? ? ? ? ? ? ?ws.OnOpen?+=?(sender,?e)?=>
? ? ? ? ? ? ? ?{
? ? ? ? ? ? ? ? ? ?isConnected?=?true;
? ? ? ? ? ? ? ? ? ?Console.WriteLine("已連接到聊天室");
? ? ? ? ? ? ? ? ? ?Console.WriteLine("輸入消息按回車發(fā)送,輸入?'exit'?退出");
? ? ? ? ? ? ? ?};
? ? ? ? ? ? ? ?//?接收消息的處理 ?
? ? ? ? ? ? ? ?ws.OnMessage?+=?(sender,?e)?=>
? ? ? ? ? ? ? ?{
? ? ? ? ? ? ? ? ? ?Console.WriteLine(e.Data);
? ? ? ? ? ? ? ?};
? ? ? ? ? ? ? ?//?發(fā)生錯誤時的處理 ?
? ? ? ? ? ? ? ?ws.OnError?+=?(sender,?e)?=>
? ? ? ? ? ? ? ?{
? ? ? ? ? ? ? ? ? ?Console.WriteLine($"錯誤:?{e.Message}");
? ? ? ? ? ? ? ?};
? ? ? ? ? ? ? ?//?連接關(guān)閉時的處理 ?
? ? ? ? ? ? ? ?ws.OnClose?+=?(sender,?e)?=>
? ? ? ? ? ? ? ?{
? ? ? ? ? ? ? ? ? ?isConnected?=?false;
? ? ? ? ? ? ? ? ? ?Console.WriteLine($"連接已關(guān)閉:?{e.Code}?{e.Reason}");
? ? ? ? ? ? ? ?};
? ? ? ? ? ? ? ?try
? ? ? ? ? ? ? ?{
? ? ? ? ? ? ? ? ? ?//?連接到服務(wù)器 ?
? ? ? ? ? ? ? ? ? ?ws.Connect();
? ? ? ? ? ? ? ? ? ?//?消息發(fā)送循環(huán) ?
? ? ? ? ? ? ? ? ? ?while?(isConnected)
? ? ? ? ? ? ? ? ? ?{
? ? ? ? ? ? ? ? ? ? ? ?string?message?=?Console.ReadLine()????"";
? ? ? ? ? ? ? ? ? ? ? ?if?(message.ToLower()?==?"exit")
? ? ? ? ? ? ? ? ? ? ? ? ? ?break;
? ? ? ? ? ? ? ? ? ? ? ?if?(!string.IsNullOrEmpty(message)?&&?ws.ReadyState?==?WebSocketState.Open)
? ? ? ? ? ? ? ? ? ? ? ?{
? ? ? ? ? ? ? ? ? ? ? ? ? ?ws.Send(message);
? ? ? ? ? ? ? ? ? ? ? ?}
? ? ? ? ? ? ? ? ? ?}
? ? ? ? ? ? ? ? ? ?//?正常關(guān)閉連接 ?
? ? ? ? ? ? ? ? ? ?if?(ws.ReadyState?==?WebSocketState.Open)
? ? ? ? ? ? ? ? ? ?{
? ? ? ? ? ? ? ? ? ? ? ?ws.Close(CloseStatusCode.Normal);
? ? ? ? ? ? ? ? ? ?}
? ? ? ? ? ? ? ?}
? ? ? ? ? ? ? ?catch?(Exception?ex)
? ? ? ? ? ? ? ?{
? ? ? ? ? ? ? ? ? ?Console.WriteLine($"發(fā)生異常:?{ex.Message}");
? ? ? ? ? ? ? ?}
? ? ? ? ? ? ? ?Console.WriteLine("按任意鍵退出...");
? ? ? ? ? ? ? ?Console.ReadKey();
? ? ? ? ? ?}
? ? ? ?}
? ?}
}
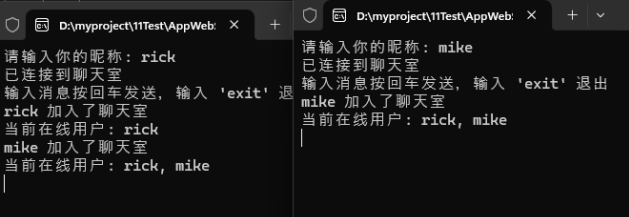
4.3 帶安全連接的服務(wù)器示例
var?wssv?=?new?WebSocketServer(4649,?true);?//?true?表示使用?SSL
wssv.SslConfiguration.ServerCertificate?=?
? ?new?X509Certificate2("/path/to/cert.pfx",?"password");
5. 高級特性
5.1 消息壓縮
//?客戶端啟用壓縮
ws.Compression?=?CompressionMethod.Deflate;
//?服務(wù)器端忽略壓縮請求
public?class?CompressIgnoredService?:?WebSocketBehavior
{
? ?public?CompressIgnoredService()
? ?{
? ? ? ?IgnoreExtensions?=?true;
? ?}
}
5.2 HTTP 代理支持
var?ws?=?new?WebSocket("ws://example.com");
ws.SetProxy("http://proxy.example.com:3128",?"username",?"password");
5.3 Cookie 處理
//?客戶端設(shè)置?Cookie
ws.SetCookie(new?Cookie("session",?"abc123"));
//?服務(wù)器端驗(yàn)證?Cookie
wssv.AddWebSocketService<ChatRoom>("/chat",?
? ?service?=>?{
? ? ? ?service.CookiesValidator?=?(req,?res)?=>?{
? ? ? ? ? ?var?sessionCookie?=?req["session"];
? ? ? ? ? ?return?sessionCookie?!=?null?&&?ValidateSession(sessionCookie.Value);
? ? ? ?};
? ?});
5.4 日志記錄
//?設(shè)置日志級別
ws.Log.Level?=?LogLevel.Debug;
//?輸出日志
ws.Log.Debug("調(diào)試信息");
ws.Log.Info("普通信息");
ws.Log.Error("錯誤信息");
6. 結(jié)語
WebSocket-sharp 提供了豐富的功能和靈活的 API,使其成為構(gòu)建實(shí)時通信應(yīng)用的理想選擇。通過本指南的示例,你可以快速開始使用 WebSocket-sharp 開發(fā)各類 WebSocket 應(yīng)用。
記住要經(jīng)常查看官方文檔以獲取最新更新和詳細(xì)信息。
https://github.com/sta/websocket-sharp
該文章在 2024/12/2 9:52:48 編輯過